Developer documentation
Logs mastering
All Lemonway_Payment extension log files are located in var/log/lemonway/
Debug mode
Debug mode depends on “enable logging” configuration.
- If enabled, logs will be more verbose and all debug details will be reported
indebug.log
. - If disabled, only errors and important information will be recorded in log
files.
API requests
All requests to the Lemonway API are logged into api.log
Payment gateway
All logs related to the payment gateway are recorded intogateway.log
Webhook
Notifications sent from Lemonway to the webhook are logged into webhook.log
Cron jobs
All logs related to cron jobs are located in cron/*
- Notifications process actions are logged into
notification_process.log
- Orders cancel actions are logged into
order_cancel.log
Notification process
All notifications that come from Lemonway through the Webhook are saved in database in the lemonway_payment_notification
table.
A cron job is configured in the extension to process these notifications every
minute:
<job name="lemonway_payment_process_notifications"
instance="Lemonway\Payment\Cron\ProcessNotifications" method="execute">
<schedule>* * * * *</schedule>
</job>
If the process of a notification encounters an error, the error message is logged
and saved in the error column of the notification entry in the database.
A notification in the error state is retried 10 times. After 10 retries, the notification is not
processed anymore and may require a manual technical investigation.
Customization
Sending a custom commission amount
If auto commission configuration is disabled, you may want to send your own
dynamically calculated commission amount.
To do so, you need to create a custom module (Foo_Bar
in the example) and add
your own DataBuilder
to the LemonwayCcGenericRequest
virtual type.
In etc/di.xml
, set up your custom DataBuilder
:
<virtualType name="LemonwayCcGenericRequest"
type="Magento\Payment\Gateway\Request\BuilderComposite">
<arguments>
<argument name="builders" xsi:type="array">
<item name="commission_amount"
xsi:type="string">Foo\Bar\Gateway\Request\CommissionAmountDataBuilder</item>
</argument>
</arguments>
</virtualType>
Then, create the class implementing the commission amount calculation logic.
The data will be automatically merged to other request data:
<?php
declare(strict_types= 1 );
namespace Foo\Bar\Gateway\Request;
use Magento\Payment\Gateway\Helper\SubjectReader;
use Magento\Payment\Gateway\Request\BuilderInterface;
/**
* Commission Amount Data Builder
*/
class CommissionAmountDataBuilder implements BuilderInterface
{
/**
* @inheritdoc
*/
public function build(array $buildSubject): array
{
// Data Object to retrieve payment and order objects if needed
$paymentDataObject = SubjectReader:: readPayment ($buildSubject);
return [
'commission_amount' => // Add your custom amount (in centime) here
];
}
}
Implementing a custom deferred capture or refund strategy
How to capture a custom order amount. In case you need to capture a custom order amount using our API, you should use the following function:
\Lemonway\Payment\Model\Webapi\GenericWebapi::execute($data)
To do so, you will need to initialize the abstractEnpoint
argument of the
GenericWebapi class with a virtual type in etc/di.xml
:
<virtualType name="MyCustomCapture"
type="Lemonway\Payment\Model\Webapi\GenericWebapi">
<arguments>
<argument name="abstractEndpoint"
xsi:type="object">Lemonway\Payment\Service\Endpoint\CapturePayment</argument>
</arguments>
</virtualType>
Then you can inject your MyCustomCapture
virtual type wherever you want.
Once it's done, you have to pass an array of parameters to the $this-myCustomCapture->execute($data)
function.
Here is an example of the $data
array content:
'website_id' => $websiteId, // Order website ID
'client_ip' => $order->getRemoteIp(), // IP of the customer who ordered
'environment' => $this->config->getEnvironment($websiteId), // Lemonway
environment (live or test)
'environment_name' => $this->config->getEnvironmentName($websiteId), // Lemonway
environment name
'total_amount' => $order->getBaseGrandTotal() * 100 , // Amount to capture (in
centime)
'transaction_id' => $payment->getParentTransactionId(), // Transaction ID
associated to the payment
Note that you can get all needed configurations from the
\\Lemonway\\Payment\\Provider\\Config
class.
For SEPA Direct Debit payments you will need to use a different endpoint and
pass an array with different data:
<virtualType name="MyCustomCapture"
type="Lemonway\Payment\Model\Webapi\GenericWebapi">
<arguments>
<argument name="abstractEndpoint"
xsi:type="object">Lemonway\Payment\Service\Endpoint\CaptureSddPayment</argument>
</arguments>
</virtualType>
'website_id' => $websiteId, // Order website ID
'client_ip' => $order->getRemoteIp(), // IP of the customer who ordered
'environment' => $this->config->getEnvironment($websiteId), // Lemonway
environment (live or test)
'environment_name' => $this->config->getEnvironmentName($websiteId), // Lemonway
environment name
'total_amount' => $order->getBaseGrandTotal() * 100 , // Amount to capture (in
centime)
'sdd_mandate_id' => $payment->getAdditionalInformation('document_id'), //
Mandate ID associated to the payment
'account_id' => $this->config->getSddAccountId($websiteId), // Lemonway account
ID
'reference' => $order->getIncrementId(), // Order reference
'comment' => "$storeName: #{$order->getIncrementId()}", // Capture comment
'autoCommission' => $this->config->isSddAutoCommissionEnabled($websiteId), //
Enable or not auto commission
How to refund a custom order amount
The customization process is the same as the “custom capture” described above.
You just need to use a different endpoint in etc/di.xml
:
<virtualType name="MyCustomRefund"
type="Lemonway\Payment\Model\Webapi\GenericWebapi">
<arguments>
<argument name="abstractEndpoint"
xsi:type="object">Lemonway\Payment\Service\Endpoint\RefundPayment</argument>
</arguments>
</virtualType>
Mirakl integration
In case the Mirakl Magento 2 Connector is installed on your Adobe Commerce
(Magento 2) application, please read the documentation below.
With the Mirakl Magento 2 Connector , when an order is created, an Observer
InitOrderItemsInvoicedAmountsObserver
excludes all Mirakl items from the invoice
amount:
/**
* Define some default invoice amounts on Mirakl orders because when we are
invoicing some order items that are not
* Mirakl offers, Magento uses some invoice amounts to build the invoice totals and
takes the order grand total
* including Mirakl offers that are not invoiced in Magento but in Mirakl platform
(by the shops).
*/
foreach ($order->getAllItems() as $item) {
/** @var \Magento\Sales\Model\Order\Item $item */
if ($item->getMiraklOfferId()) {
$order->setSubtotalInvoiced($order->getSubtotalInvoiced() + $item-
>getRowTotal());
$order->setBaseSubtotalInvoiced($order->getBaseSubtotalInvoiced() +
$item->getBaseRowTotal());
$order->setTaxInvoiced($order->getTaxInvoiced() + $item-
>getTaxAmount());
$order->setBaseTaxInvoiced($order->getBaseTaxInvoiced() + $item-
>getBaseTaxAmount());
}
}
This is done this way because Adobe Commerce (Magento) orders are exported and split into several orders for each seller inside the Mirakl platform. Then, if a seller cannot ship an item for any reason, it can be canceled in Mirakl Platform, but this information won’t be updated in the Adobe Commerce (Magento) order.
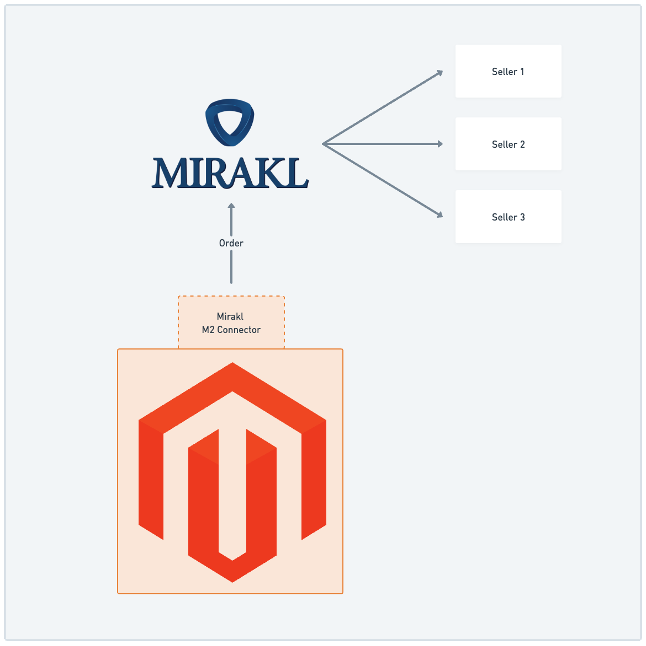
With this workflow, you cannot fully invoice an order from the Adobe Commerce (Magento) back office. As the Lemonway_Payment
the extension needs a full invoice to trigger a deferred capture, you will need to customize your application to capture the correct amount once all items are processed (confirmed or canceled) by the
sellers inside the Mirakl platform.
To do so, you will need to call the Mirakl API to get updates on the items after the order has been exported to the Mirakl platform. Please refer to the official Mirakl API documentation (provided with your Mirakl account).
Once you know that all the items of an order have been processed inside the Mirakl platform, you can trigger a custom deferred capture by following our documentation.
Updated about 1 month ago